The Menu API allows you to upload or update menus for a specific store and location, track processing status in real time, receive webhook notifications about menu updates, and pull the latest version of a menu from a provider.
🧭 Menu Management Options
You can manage menus using two main approaches depending on your needs and scale. The Admin Dashboard is ideal for manually entering menus during initial setup, testing, or for occasional changes by non-technical users. API Integration is best suited for high-volume operations, multi-location updates, or when syncing with internal systems like a CMS or POS to automate menu creation, updates, and synchronization across platforms.
Tip: You can start manually and switch to API-based updates later.
🔐 Prerequisites
Before using the Menu API, make sure you have:
- Authorization Token – required in all requests
- Location ID and Store ID – represent the physical and virtual endpoints for menu delivery, respectively. The location refers to where the menu will be used (e.g., a restaurant branch), and the store represents the marketplace-facing unit that customers interact with. Both must be created in the system before any menu can be uploaded.
Menus are always linked to both a location and a store. These must exist before uploading menus.
🛠 Menu Creation via API
Endpoint: POST /menus
Creates or updates a menu for a given location and store.
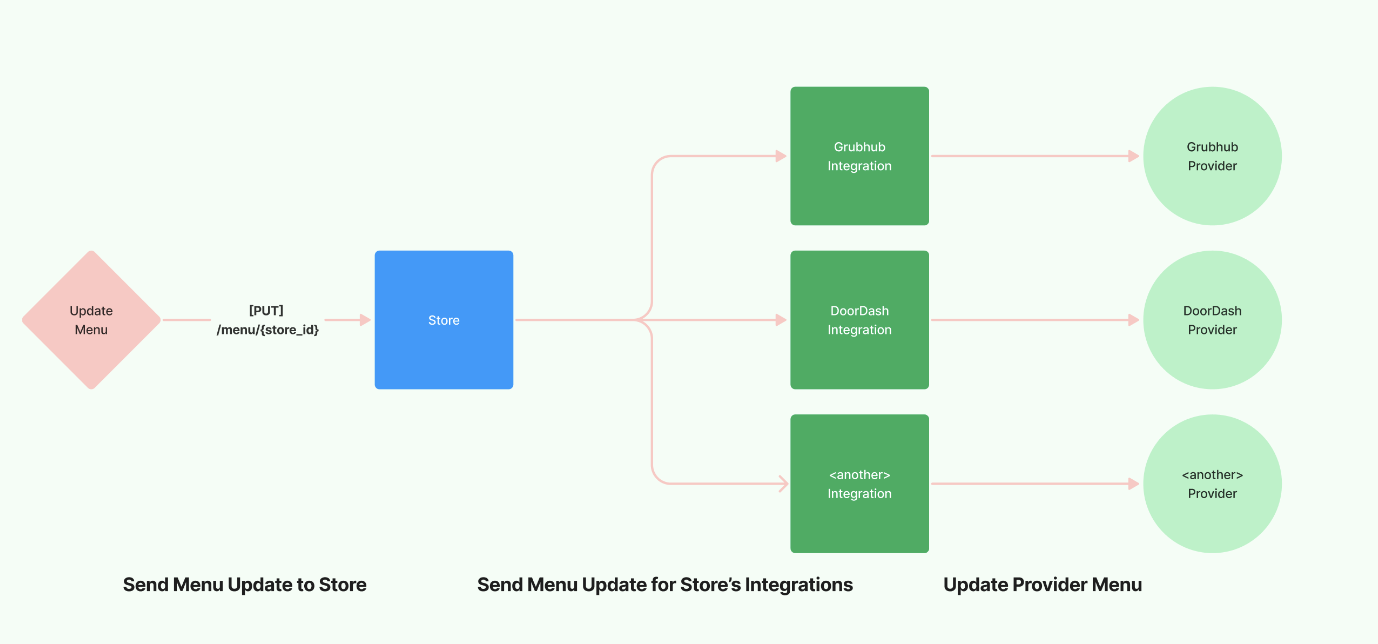
Example Request
POST /menus
{
"location_id": "location_123",
"store_id": "store_456",
"menu_items": [
{
"id": "item_001",
"name": "Margherita Pizza",
"description": "Classic pizza with tomatoes, mozzarella, and basil",
"price": 10.99,
"category": "Pizza",
"available": true
},
{
"id": "item_002",
"name": "Spaghetti Carbonara",
"description": "Pasta with pancetta and creamy sauce",
"price": 12.99,
"category": "Pasta",
"available": true
}
]
}
Example Response
{
"menu_id": "menu_789",
"status": "processing",
"message": "Menu received and is being processed."
}
Price format: Use decimal numbers (e.g.,
10.99
), not cents.
🧱 Menu Structure
A menu in KitchenHub is organized as a layered system of elements that together define what a restaurant offers and how it’s presented across delivery platforms. The structure is designed to give maximum flexibility, allowing for reusable components, dynamic availability, and scalable control across multiple providers.
🗂 Categories
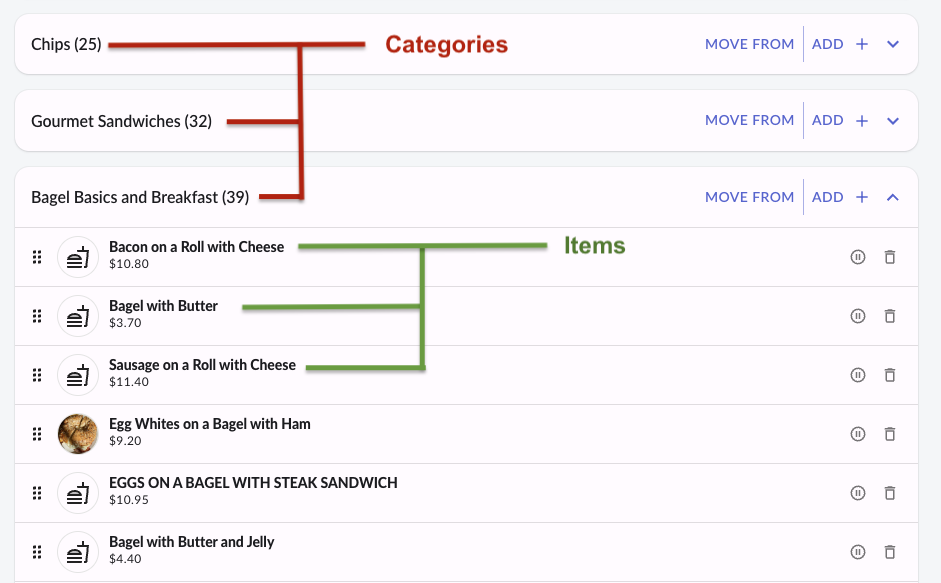
Categories group related items under clear sections like “Burgers,” “Sides,” or “Breakfast Specials.” Categories not only organize the menu visually, but also help define availability and user flow.
You can pause categories, schedule their availability, and reorder them as needed. An item can belong to multiple categories at the same time — for example, “French Fries” may appear in both “Combos” and “Snacks.”
🍽 Items
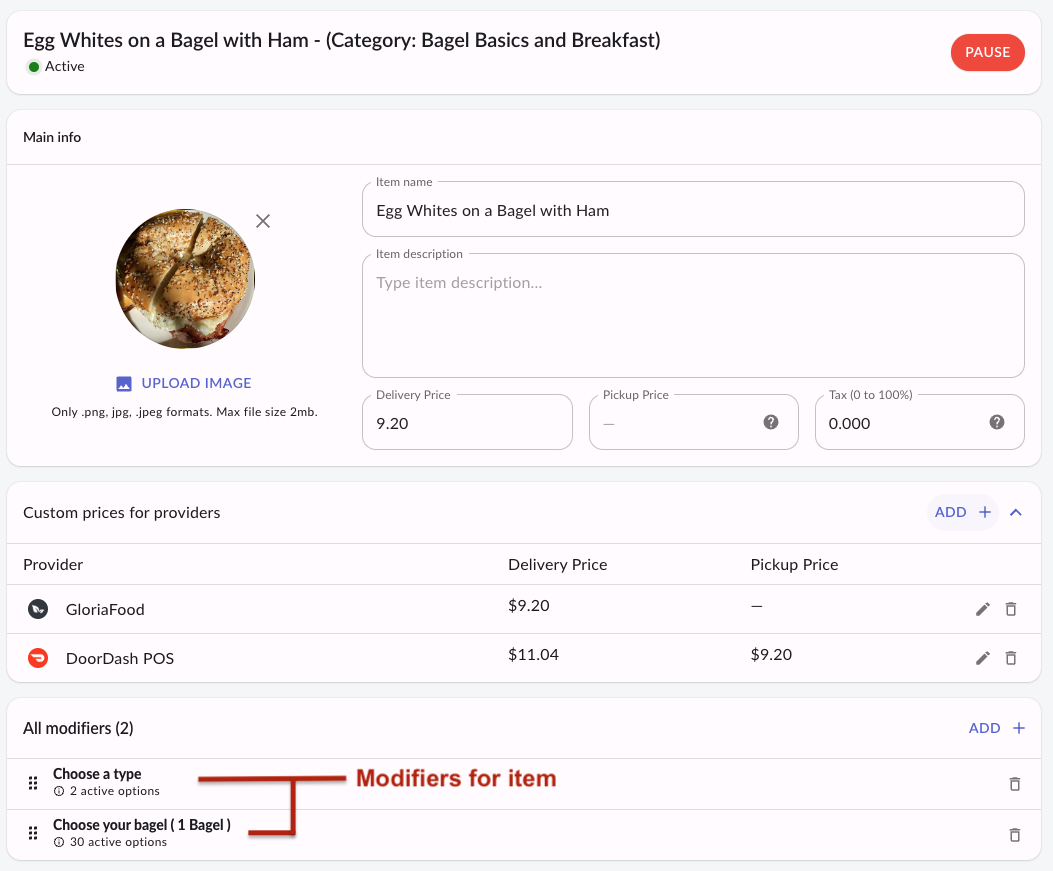
Items are the individual dishes or products that customers can order. Each item contains a name, price, image, and optionally a description. You can define custom prices per provider, and control visibility using availability rules or by pausing the item.
Items are always placed in one or more categories. This allows you to reuse the same item in different menu contexts without duplicating its content or logic.
🧩 Modifiers
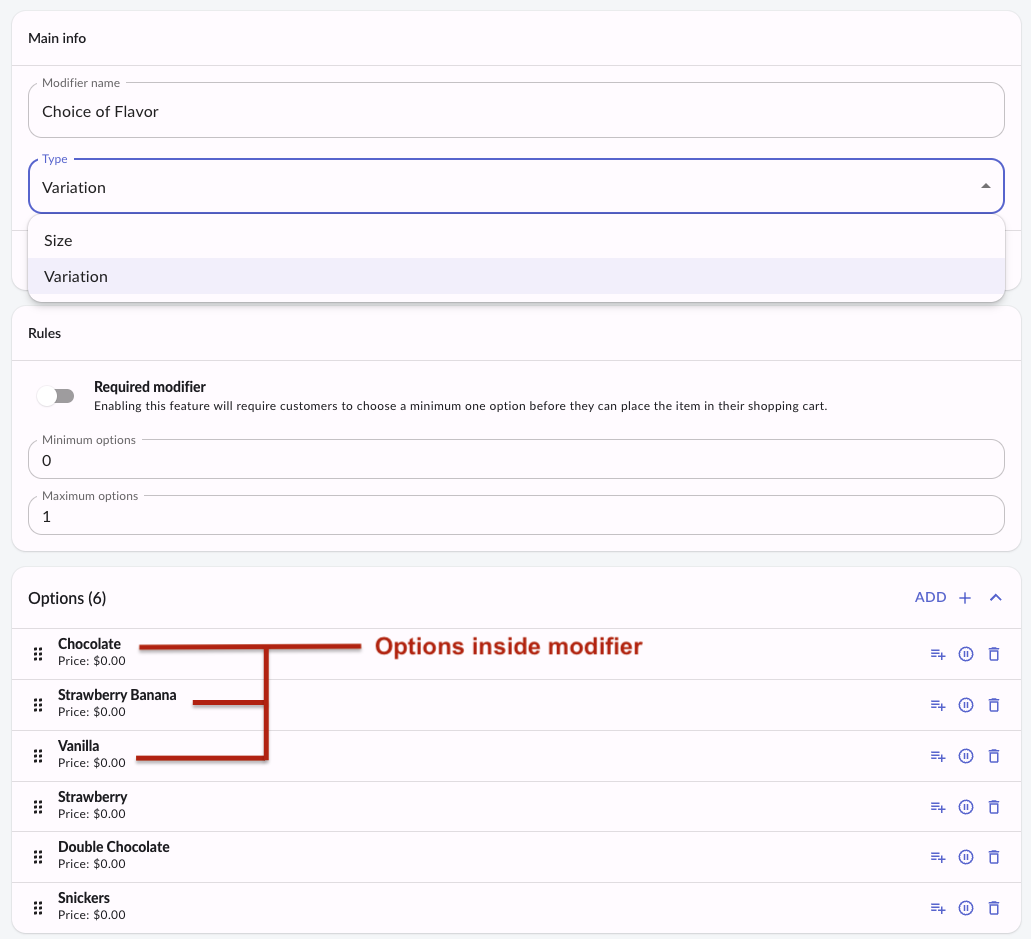
Modifiers let customers personalize their order — for instance, choosing a side dish, selecting a sauce, or picking a pizza crust. A modifier belongs to one or more items and defines a set of options the customer can select from.
Modifiers can be reused across multiple items. You can make them required or optional, set min/max selection limits, and customize their behavior to fit the use case (e.g., forcing the customer to pick one size).
🔘 Options
Options are the actual choices within a modifier — for example, “Small,” “Medium,” or “Large” for a drink, or different flavors for a dessert.
Options can be paused and scheduled just like items or categories. Importantly, they come in two types:
- Size — typically exclusive selections that define how the item is portioned or priced.
- Variation — more flexible, often used for extras, add-ons, or flavor selections.
The type of option affects how it is interpreted and displayed in third-party marketplaces, so choosing the right one ensures better integration and user experience.
⏰ Availability
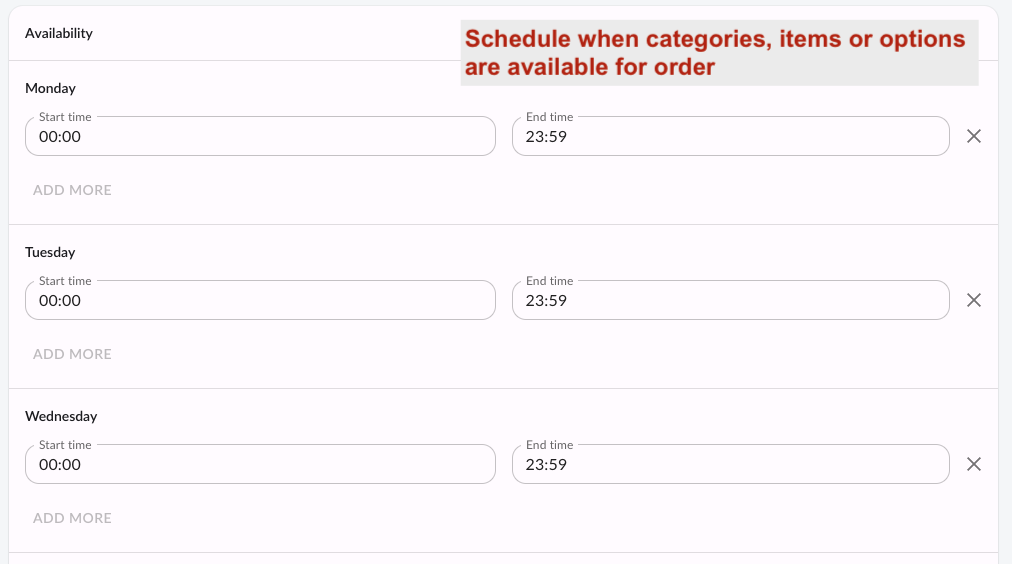
Availability rules can be set for categories, items, and individual options. This gives you granular control over what customers see and when — for example, breakfast items available only in the morning, or seasonal toppings shown only on weekends.
You can schedule availability by day of the week and time ranges, apply different rules at each level, and pause any component without deleting it.
🔗 How It All Connects
- A menu contains categories
- Each category holds multiple items
- Each item can appear in several categories
- Modifiers are assigned to one or more items
- Each modifier contains options, which may be of type size or variation
- Availability and pause status can be configured on categories, items, modifiers, and options
This structure lets restaurants create powerful, flexible menus that match the real-world complexity of operations, while maintaining a clean, reusable, and scalable backend setup.
🧩 Nested Modifiers
Modifiers can be organized in a nested structure to support complex menu configurations (e.g., pizza > size > toppings > extra cheese).
🔨 How to Use
- Use the
partner_modifiers
field inside an Option to attach nested modifiers. - Each nested modifier must be defined first as a Modifier object.
- You can reference that modifier inside an option to create a tree-like structure.
⟳ Example Nesting Logic
- Create a Modifier (e.g., "Size").
- Inside the Option linked to that modifier, use
partner_modifiers
to define further nested modifiers (e.g., "Extra Toppings"). - Continue up to 3 levels deep.
Nesting Limit: Maximum nesting depth is 3. Deeper nesting is not supported.
✅ Provider Compatibility
Provider | Nested Modifiers Support |
---|---|
DoorDash | ✅ Supported |
UberEats | ✅ Supported |
GrubHub | ✅ Supported |
Others | ❌ Not Supported nested modifiers will be flattened into the item level |
💸 Price Customization per Provider
You can set different prices for the same item depending on the provider. This allows flexibility for delivery fees, promotions, or platform-specific pricing.
🔧 Setup
In the Item object:
- Use the
price
field for the base price - Use the optional
prices_customization
object to define provider-specific pricing
{
"id": "item_001",
"name": "Margherita Pizza",
"price": 10.99,
"prices_customization": [
{
"provider_id": "ubereats",
"price": 11.49,
"original_price": 10.99
},
{
"provider_id": "doordash_pos",
"price": 11.99,
"original_price": 10.99
}
]
}
📝 Field Descriptions
- price: Default price (used if no customization is provided)
- provider_id: ID of the external platform (e.g.,
ubereats
,doordash_pos
) - price (in customization): Price including delivery
- original_price: Price for pickup.
Only available for
doordash_pos
provider.
📋 Menu-to-Order Mapping
Some third-party providers require that incoming orders reference the same item IDs used in the uploaded menu. This process is known as menu mapping and is essential for partners who tightly couple their ordering flow with the menu structure.
⟳ How It Works
- Automatic Mapping: Once your menu is uploaded to the provider through KitchenHub, mapping begins automatically — no extra steps are needed.
- Item Identity: To enable mapping, ensure that each item in your menu uses a stable and consistent ID from your internal system.
Important: Use the
partner_id
field in each nested menu object (items, modifiers, options) to supply the ID from your system. This ensures the orders can be correctly matched to your menu items.
📊 Checking Menu Status
Endpoint: GET /menus/{menu_id}/status
Checks whether the uploaded menu has been processed by KitchenHub and pushed to connected providers.
Example Request
GET /menus/menu_789/status HTTP/1.1
Host: api.kitchenhub.com
Authorization: Bearer YOUR_AUTH_TOKEN
Example Response
{
"menu_id": "menu_789",
"status": "completed",
"provider_statuses": [
{
"provider": "UberEats",
"status": "completed",
"updated_at": "2025-03-22T12:14:09Z"
},
{
"provider": "DoorDash",
"status": "completed",
"updated_at": "2025-03-22T12:14:35Z"
}
]
}
❓ FAQ
Q: Why does my menu not appear immediately in the provider's system?
Some providers have internal verification workflows that introduce a delay after menu submission. This means that even after a successful upload via KitchenHub, your menu may take additional time to appear live due to provider-side review or approval processes.
Q: What happens if my menu has structural issues?
The Menu API performs strict validation on the structure of your menu. Ensure that the format strictly matches the API schema — incorrect field names, missing required fields, or malformed objects will cause the request to fail.
Q: Can partner_id
values be duplicated?
No. All partner_id
values (for items, modifiers, options) must be unique within the menu. Duplicate partner_id
s will result in validation errors during upload.
Q: Will the menu appear exactly the same on all providers?
Not necessarily. Each provider has its own formatting, rules, and limitations. While we standardize as much as possible, some visual or structural differences may occur after sync.
Q: Do all providers support menu uploads?
No. Some providers do not allow menu imports via API. The list of supported providers is available here.
Q: Can I manage multiple menus for a single location?
KitchenHub does not support multiple separate menus per location. However, our API provides flexible visibility settings at the item and category level, allowing you to simulate multiple menus by toggling availability.
Q: Can I update only one item or category in the menu?
No. The KitchenHub API is designed to replace the entire menu when any change is made. For example, if you want to pause a single item or update its availability, you must resend the full menu including that change. Partial updates are not supported.
Q: Are images always re-uploaded with the menu?
No. To speed up processing, we only re-upload images if they have changed. If the image URLs are unchanged, they will be reused and not reprocessed.
Q: Are there any requirements for images in the menu?
Yes. Images must be hosted at publicly accessible URLs. Recommended image formats are JPG or PNG with a minimum resolution of 1024 pixels and file size up to 2MB. Large or unoptimized images may slow down sync performance.
Q: Why are my images not visible right after pushing the menu?
Image processing is asynchronous. After a menu is uploaded, images may appear with a short delay. To avoid broken image links, make sure your image URLs remain accessible at all times.
Menu Model
Top-level of the menu object.
Property | Type | Nullable | Description |
---|---|---|---|
id | string | No | The ID of the menu on the Kitchenhub side |
store_id | string | No | The ID of the store that the menu is connected |
menu | Object | No | General info about the menu |
categories | Array of objects | No | Menu categories and their properties |
items | Array of objects | No | Menu items and their properties |
modifiers | Array of objects | No | Modifier questions for items |
options | Array of objects | No | Options for modifiers |
availabilities | Array of objects | No | Schedules of availabilities for categories, items, modifiers, and options |
Menu object properties
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the menu on the partner side |
name | string | No | Menu name. |
description | string | Yes | Menu description. |
media_url | string | Yes | URL of the image of the menu. |
partner_categories | Array of strings | Yes | A list of category IDs on the partner side belongs to this menu. Categories will be displayed in the same order they are listed here. |
Category object
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the category on the partner side. |
title | string | No | Category name. |
description | string | Yes | Category description. |
is_deactivated | boolean | Yes | If it's true then the whole category will be disabled for ordering. |
deactivation_start_date | date-time | Yes | Date since the category is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ ).You can use it for scheduled deactivation. |
deactivation_end_date | date-time | Yes | Date till the category is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ )You can use it for scheduled enabling of the category. |
partner_items | Array of strings | Yes | A list of item IDs on the partner side belongs to this category. Items will be displayed in the same order they are listed here. |
partner_availability | String | Yes | Availability schedule ID on the partner side. Can be used to hide the category for specific times of the day, or days of the week. Widely used to build launch or other special menus. |
Item object
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the item on the partner side. |
name | string | No | Item name. |
description | string | Yes | Item description. |
internal_notes | string | Yes | Is used to display additional information for items (for example preparation instructions) on the order level when they are ingested into a restaurant. Isn't visible to end customers. |
is_deactivated | boolean | Yes | If it's true then the item will be disabled for ordering. |
deactivation_start_date | date-time | Yes | Date since the item is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ ).You can use it for scheduled deactivation. |
deactivation_end_date | date-time | Yes | Date till the item is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ )You can use it for scheduled enabling of the item. |
media_url | string | Yes | The image you have selected will be transformed to fit provider requirements. We recommend to keep at least 1024 px each side. If provider expects specific aspect-ratio, white borders can be added to fit this requirement. |
price | decimal | No | Price of the item. This field should be a positive number |
original_price | decimal | Yes | The cost of the item for pickup. This price does not include delivery charges. This field should be a positive number. |
tax_rate | decimal | Yes | Extra tax which may be added to some items on top of regular sales tax. Must be between 0 and 1 |
min_amount | integer | Yes | Set the rule of the minimum quantity of the item customers have to order. If null then there are no restrictions |
max_amount | integer | Yes | Set the rule of the maximum quantity of the item customers can order. If null then there are no restrictions |
partner_modifiers | Array of strings | No | A list of modifier IDs on the partner side belongs to this Item. Modifiers will be displayed in the same order they are listed here. |
partner_availability | String | Yes | Availability schedule ID on the partner side. Can be used to hide the item for specific times of the day, or days of the week. Widely used to build launch or other special menus. |
prices_customization | Dict[str, CustomPriceSchema] | No | Custom price information for selected providers. This field can be skipped, but cannot contain a null value. Contains mapping of provider_id (str) to prices information (CustomPriceSchema). Supports providers with a full menu support protocol. Use the provider_id from the Provider Integrations table here, ensuring the "Menu API" column indicates full support. Note that the original_price field is currently supported only by Doordash provider (Provider ID: doordash_pos). Usage Example: [ { "doordash_pos": { "price": 10, "original_price": 8 } } ]CustomPriceSchema explained further |
CustomPriceSchema
Property | Type | Nullable | Description |
---|---|---|---|
price | decimal | No | The price includes delivery charges. This is a required field and must be a positive number. |
original_price | decimal | Yes | The price for pickup. This price does not include delivery charges. This field is used only by DoorDash and should be a positive number. This field is optional. This field is nullable and can be 0. The processing of this case depends on the provider. Note that the original_price field is currently supported only by Doordash provider (Provider ID: doordash_pos). |
Modifier object
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the modifier on the partner side. |
name | string | No | Modifier name. |
type | string | No | Can be size or variation .If it is size then only one option can be chosen, and it means that min_options = 1 max_options = 1 has to be set.If it's variation you can set min_options andmax_options as you need. |
is_required | boolean | Yes | If it's true then an end customer has to choose at least one option from the list of options connected to this modifier. |
min_options | integer | No | Set the rule of the minimum quantity of the options customers have to choose. Work together with max_options |
max_options | integer | No | Set the rule of the maximum quantity of the options customers have to choose. Examples of how it should be used together with min_options :- Choose 1 from many: min_options = 1 max_options = 1 - Choose any up to 10 : min_options = 1 max_options = 10 - Choose 3 options: min_options = 3 max_options = 3 |
partner_options | Array of strings | No | A list of options IDs on the partner side belongs to this modifier. Options will be displayed in the same order they are listed here. |
default_options | Array of strings | Yes | Set which options from the list will be chosen by default for customers. It can be one or multiple options. Check if it fits right with the max_options parameter. |
Option object
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the option on the partner side. |
name | string | No | Option name. |
description | string | Yes | Item description. |
is_deactivated | boolean | Yes | If it's true then the item will be disabled for ordering. |
deactivation_start_date | date-time | Yes | Date since the option is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ ).You can use it for scheduled deactivation. |
deactivation_end_date | date-time | Yes | Date till the option is deactivated (UTC time in format year-month-dayThour:minute:second.msecZ )You can use it for scheduled enabling of the option. |
price | decimal | Yes | Price of the option, if null then the option is free. |
original_price | decimal | Yes | The cost of this option before discounts and promotions. Can be used as a reference price for statistics. Isn't visible to end customers. |
tax_rate | decimal | Yes | The extra tax may be added to some options despite regular sales tax. Must be between 0 and 1. |
partner_modifiers | Array of strings | Yes | Extra modifiers can be added to the option. You can add to an item maximum 3-level of nested modifiers. |
Availability object
Used to assign specific time periods of availability to items and categories. (not to be confused with business hours or opening hours). For example: Breakfast Burrito from 9:00 AM to 11:45 AM
Property | Type | Nullable | Description |
---|---|---|---|
partner_id | string | No | The ID of the category on the partner side. |
rules | array of objects | No | See bellow |
Rule object
Property | Type | Nullable | Description |
---|---|---|---|
days_of_week | array of strings | No | List of days of the week (MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY) |
start_time | string | No | Time after which item is available in 24-hour HH:MM:SS.MS format |
end_time | string | No | Time after which item is not available in 24-hour HH:MM:SS.MS format |
Menu example
{
"menu": {
"partner_id": "Menu",
"name": "Menu",
"description": null,
"media_url": null,
"partner_categories": [
"Paninis",
"Breakfast_Omelettes",
"Flagels",
"From_the_Bakery",
"Fruit_Smoothie_Secti",
"Beverages"
]
},
"categories": [
{
"partner_id": "b914a949-50fb-4669-a0ff-1115f83bd1c2",
"title": "Dinner Combo",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"partner_items": [
"d2024709-5c8b-44d2-b228-639704ca9df7"
],
"partner_availability": null
},
{
"partner_id": "Fruit_Smoothie_Secti",
"title": "Fruit Smoothie Section",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Berry_Blast",
"Summer_Love",
"Banana_split",
"The_East_Villager",
"The_Stuy_Towner",
"Create_Your_Own"
],
"partner_availability": null
},
{
"partner_id": "Flagels",
"title": "Flat Bagels",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Flagels_49908",
"Dozen_Fagels"
],
"partner_availability": null
},
{
"partner_id": "Breakfast_Omelettes",
"title": "Breakfast Omelettes",
"description": null,
"is_deactivated": true,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Eggs_and_Vegetable_O",
"Leo_Omelette",
"Greek_Omelette",
"Western_Omelette"
],
"partner_availability": null
},
{
"partner_id": "From_the_Bakery",
"title": "From the Bakery",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Muffin",
"Raspberry_Cigar",
"Cinnamon_Bun_Danish",
"Linzer_Tart",
"Croissant",
"Turnover",
"Chocolate_Chip_Brown"
],
"partner_availability": null
},
{
"partner_id": "Paninis",
"title": "Paninis",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"The_Dylan_Panni",
"The_Michelle_Panini",
"The_Danny_Boy_Panini"
],
"partner_availability": null
},
{
"partner_id": "Beverages",
"title": "Beverages",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Can_of_Soda",
"Bottled_Soda_(20_oz)",
"Tropicana_Juice",
"Quart_of_Tropicana_J"
],
"partner_availability": null
}
],
"items": [
{
"partner_id": "d2024709-5c8b-44d2-b228-639704ca9df7",
"name": "Vegetarian Combo",
"description": "Vegetarian Combo",
"internal_notes": "",
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 20.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"60217cba-b454-4999-9a6f-fb41ef1cbb23",
"b5b9d68b-b215-44b4-8a27-0d60ff5bf0f5"
],
"tax_rate": 0.0
},
{
"partner_id": "Raspberry_Cigar",
"name": "Raspberry Cigar",
"description": "A flaky pastry filled with raspberry preserves that creates a deliciously sweet and tangy dessert. It's the perfect treat to enjoy with a cup of coffee or tea.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Chocolate_Chip_Brown",
"name": "Chocolate Chip Brownie",
"description": "Indulge in our decadent Chocolate Chip Brownie, made with rich cocoa and loaded with delicious chocolate chips for the ultimate chocolatey treat. Perfectly baked to perfection, this brownie is soft, chewy, and oh-so-satisfying!",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 7.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_Michelle_Panini",
"name": "The Michelle Panini",
"description": "Indulge in The Michelle Panini, a culinary masterpiece that showcases a delightful combination of premium ingredients. From the perfectly grilled bread to the melty cheeses, savory meats, and vibrant flavors of fresh ingredients, each bite of this delectable creation is a true delight that will transport your taste buds to a realm of pure satisfaction. ",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Bottled_Soda_(20_oz)",
"name": "Bottled Soda (20 oz)",
"description": "Indulge in the ultimate thirst-quenching experience with our 20 oz Bottled Soda, where a generous serving of your favorite carbonated beverage awaits. Savor every effervescent sip as it tantalizes your taste buds and provides a refreshing escape from the everyday.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 5.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_drink"
],
"tax_rate": 0.08875
},
{
"partner_id": "Western_Omelette",
"name": "Western Omelette",
"description": "The Western Omelette is made with three eggs and filled with diced ham, peppers, onions, and served with home fries and a buttered bagel; it is a classic breakfast dish with a satisfying blend of flavors and textures.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 2,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Linzer_Tart",
"name": "Linzer Tart",
"description": "The Linzer Tart is a traditional Austrian pastry made with a nutty shortbread crust and filled with raspberry preserves, making for a sweet and delicious treat. It's perfect for anyone with a sweet tooth looking for a classic dessert option.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Muffin",
"name": "Muffin",
"description": "A freshly baked and moist muffin, bursting with flavor and perfect for a quick breakfast or snack.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Berry_Blast",
"name": "Berry Blast",
"description": "Berry Blast is a delicious and refreshing smoothie made with a blend of mixed berries, providing a burst of fruity flavor and healthy nutrients in every sip.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "The_Stuy_Towner",
"name": "The Stuy Towner",
"description": "The Stuy Towner smoothie is a delicious blend of creamy peanut butter, ripe banana, and decadent chocolate syrup, making for a satisfying and indulgent treat. Perfect for a quick and tasty breakfast on the go or a midday pick-me-up.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Summer_Love",
"name": "Summer Love",
"description": " is a refreshing blend of sweet strawberries, tropical pineapple, and juicy mango, perfect for a summer day. This drink is sure to transport your taste buds to a tropical paradise with every sip.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Tropicana_Juice",
"name": "Tropicana Juice",
"description": "Tropicana Juice is a refreshing and flavorful beverage made from high-quality fruits that provides essential vitamins and nutrients.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 5.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Banana_split",
"name": "Banana split",
"description": "The Banana Split smoothie is a delightful blend of sweet strawberries, ripe bananas, and rich chocolate syrup, creating a perfectly balanced and indulgent treat.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Leo_Omelette",
"name": "Leo Omelette ",
"description": "The Leo Omelette is a savory breakfast dish made with lox, eggs, and onions, served with home fries and a bagel or bialy. It's a classic combination that is both satisfying and delicious.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Create_Your_Own",
"name": "Create Your Own",
"description": "The Create Your Own smoothie allows you to select three toppings of your choice to create a personalized and delicious blend of flavors.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.59,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Turnover",
"name": "Turnover",
"description": "This delectable pastry is a sweet treat that combines soft and sweet apples with a flaky pastry, baked until golden and finished off with a delicious glaze.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Greek_Omelette",
"name": "Greek Omelette",
"description": "The Greek Omelette is a breakfast dish made with 3 eggs, feta cheese, and caramelized onions, served with home fries and a buttered bagel or bialy, and may be a savory option for those looking to start their day with a hearty meal.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Dozen_Fagels",
"name": "Dozen Flat Bagels",
"description": "A dozen of our deliciously chewy flat bagels, perfect for sharing with friends and family for breakfast or brunch. Choose from a variety of flavors including plain, sesame, poppy seed, and everything.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 48.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Eggs_and_Vegetable_O",
"name": "Vegetable Omelette ",
"description": "This dish features a fluffy three-egg omelette filled with nutritious vegetables, accompanied by crispy home fries and a choice of bagel or bialy.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 17.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Croissant",
"name": "Croissant",
"description": "A classic pastry that is perfect for breakfast or a snack, the buttery and flaky croissant can be enjoyed on its own or paired with your choice of butter or jam for added flavor.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Quart_of_Tropicana_J",
"name": "Quart of Tropicana Juice",
"description": "A refreshing and deliciously healthy way to quench your thirst, a quart of Tropicana juice is perfect for sharing with family and friends. Made from 100% pure and natural fruits, it's a great source of vitamins and minerals to help you stay energized throughout the day.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 8.65,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Flagels_49908",
"name": "Flat Bagels",
"description": "Flat bagels are an alternative to the traditional bagel that is thinner and wider with a smaller hole, making it ideal for toasting and sandwich making.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 4.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_Danny_Boy_Panini",
"name": "The Danny Boy Panini",
"description": "A culinary masterpiece crafted with care. This delectable creation features a symphony of premium ingredients, perfectly grilled bread, and a harmonious blend of savory and vibrant flavors, delivering a memorable and satisfying experience that will leave you longing for another delightful bite.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Can_of_Soda",
"name": "Can of Soda",
"description": "\\nQuench your thirst and savor the simple pleasure of an ice-cold can of your favorite soda, offering a crisp and satisfying sip that provides a delightful burst of flavor and a moment of pure refreshment.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 3.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_drink"
],
"tax_rate": 0.08875
},
{
"partner_id": "Cinnamon_Bun_Danish",
"name": "Cinnamon Bun Danish",
"description": "The Cinnamon Bun Danish is a delectable pastry featuring a flaky, buttery dough swirled with cinnamon sugar and drizzled with a sweet glaze, perfect for a breakfast treat or midday snack.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_East_Villager",
"name": "The East Villager",
"description": "The East Villager smoothie is a delightful blend of sweet blueberries, tropical pineapple, and creamy banana, creating a perfectly balanced and refreshing drink. It's a great way to start your day or give you a midday energy boost!",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "The_Dylan_Panni",
"name": "The Dylan Panni",
"description": "\\nExperience culinary perfection with The Dylan Panni, a mouthwatering creation that combines layers of succulent meats, cheeses, and fresh ingredients nestled between perfectly grilled bread, offering a harmonious symphony of flavors and textures that will leave you enchanted with every delightful bite.\\n\\n\\n\\n\\n",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
}
],
"modifiers": [
{
"partner_id": "60217cba-b454-4999-9a6f-fb41ef1cbb23",
"name": "Main Dish",
"is_required": false,
"min_options": 0,
"max_options": 1,
"default_options": [],
"partner_options": [
"47ca2d01-2751-4cc6-904c-c75029ba4ba1_10.0"
],
"type": "variation"
},
{
"partner_id": "6ade3193-5c92-4ead-82a3-f79d059de298",
"name": "Cheese Type",
"is_required": false,
"min_options": 0,
"max_options": 1,
"default_options": [],
"partner_options": [
"cc5e12b5-2f1c-45d2-809c-eee883dc8774_1.0",
"1bbc0ac1-b67e-4226-9b29-be18d13955fd_1.5"
],
"type": "variation"
},
{
"partner_id": "e0b53022-c908-4770-acc7-8fa8e863a8b1",
"name": "Cheese Quantity",
"is_required": false,
"min_options": 0,
"max_options": 1,
"default_options": [],
"partner_options": [
"48d5ea4b-76e5-4c94-84bb-8d0c89a50e3c_0.0",
"8b24dcd7-6213-4c3b-b1d1-24b71ebdc6ba_0.0"
],
"type": "variation"
},
{
"partner_id": "b5b9d68b-b215-44b4-8a27-0d60ff5bf0f5",
"name": "Vegetable Salad",
"is_required": false,
"min_options": 0,
"max_options": 1,
"default_options": [],
"partner_options": [
"3d3bf161-1e48-4646-9244-81c8ec01f6ec_3.0",
"b914a686-1de9-40a6-8e0f-4a25f2b0439c_5.0"
],
"type": "variation"
},
{
"partner_id": "Choose_your_drink",
"name": "Choose your drink",
"is_required": false,
"min_options": 1,
"max_options": 1,
"default_options": [],
"partner_options": [
"Cola",
"Diet-Cola"
],
"type": "variation"
},
{
"partner_id": "Choose_your_quantity",
"name": "Choose your quantity",
"is_required": false,
"min_options": 1,
"max_options": 1,
"default_options": [],
"partner_options": [
"16_oz",
"24_oz"
],
"type": "variation"
}
],
"options": [
{
"partner_id": "47ca2d01-2751-4cc6-904c-c75029ba4ba1_10.0",
"name": "Vegetarian Burger",
"price": 10.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": [
"6ade3193-5c92-4ead-82a3-f79d059de298"
]
},
{
"partner_id": "cc5e12b5-2f1c-45d2-809c-eee883dc8774_1.0",
"name": "Cheddar",
"price": 1.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": [
"e0b53022-c908-4770-acc7-8fa8e863a8b1"
]
},
{
"partner_id": "1bbc0ac1-b67e-4226-9b29-be18d13955fd_1.5",
"name": "Swiss",
"price": 1.5,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": [
"e0b53022-c908-4770-acc7-8fa8e863a8b1"
]
},
{
"partner_id": "48d5ea4b-76e5-4c94-84bb-8d0c89a50e3c_0.0",
"name": "Single",
"price": 3.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "8b24dcd7-6213-4c3b-b1d1-24b71ebdc6ba_0.0",
"name": "Double",
"price": 3.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "3d3bf161-1e48-4646-9244-81c8ec01f6ec_3.0",
"name": "Small",
"price": 3.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "b914a686-1de9-40a6-8e0f-4a25f2b0439c_5.0",
"name": "large",
"price": 5.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "24_oz",
"name": "24_oz",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "16_oz",
"name": "16_oz",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "Cola",
"name": "Cola",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
},
{
"partner_id": "Diet-Cola",
"name": "Diet-Cola",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_modifiers": []
}
],
"availabilities": []
}
{
"menu": {
"partner_id": "Menu",
"name": "Menu",
"description": null,
"media_url": null,
"partner_categories": [
"Paninis",
"Breakfast_Omelettes",
"Flagels",
"From_the_Bakery",
"Fruit_Smoothie_Secti",
"Beverages"
]
},
"categories": [
{
"partner_id": "Fruit_Smoothie_Secti",
"title": "Fruit Smoothie Section",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Berry_Blast",
"Summer_Love",
"Banana_split",
"The_East_Villager",
"The_Stuy_Towner",
"Create_Your_Own"
],
"partner_availability": null
},
{
"partner_id": "Flagels",
"title": "Flat Bagels",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Flagels_49908",
"Dozen_Fagels"
],
"partner_availability": null
},
{
"partner_id": "Breakfast_Omelettes",
"title": "Breakfast Omelettes",
"description": null,
"is_deactivated": true,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Eggs_and_Vegetable_O",
"Leo_Omelette",
"Greek_Omelette",
"Western_Omelette"
],
"partner_availability": null
},
{
"partner_id": "From_the_Bakery",
"title": "From the Bakery",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Muffin",
"Raspberry_Cigar",
"Cinnamon_Bun_Danish",
"Linzer_Tart",
"Croissant",
"Turnover",
"Chocolate_Chip_Brown"
],
"partner_availability": null
},
{
"partner_id": "Paninis",
"title": "Paninis",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"The_Dylan_Panni",
"The_Michelle_Panini",
"The_Danny_Boy_Panini"
],
"partner_availability": null
},
{
"partner_id": "Beverages",
"title": "Beverages",
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"partner_items": [
"Can_of_Soda",
"Bottled_Soda_(20_oz)",
"Tropicana_Juice",
"Quart_of_Tropicana_J"
],
"partner_availability": null
}
],
"items": [
{
"partner_id": "Raspberry_Cigar",
"name": "Raspberry Cigar",
"description": "A flaky pastry filled with raspberry preserves that creates a deliciously sweet and tangy dessert. It's the perfect treat to enjoy with a cup of coffee or tea.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Chocolate_Chip_Brown",
"name": "Chocolate Chip Brownie",
"description": "Indulge in our decadent Chocolate Chip Brownie, made with rich cocoa and loaded with delicious chocolate chips for the ultimate chocolatey treat. Perfectly baked to perfection, this brownie is soft, chewy, and oh-so-satisfying!",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 7.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_Michelle_Panini",
"name": "The Michelle Panini",
"description": "Indulge in The Michelle Panini, a culinary masterpiece that showcases a delightful combination of premium ingredients. From the perfectly grilled bread to the melty cheeses, savory meats, and vibrant flavors of fresh ingredients, each bite of this delectable creation is a true delight that will transport your taste buds to a realm of pure satisfaction. ",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Bottled_Soda_(20_oz)",
"name": "Bottled Soda (20 oz)",
"description": "Indulge in the ultimate thirst-quenching experience with our 20 oz Bottled Soda, where a generous serving of your favorite carbonated beverage awaits. Savor every effervescent sip as it tantalizes your taste buds and provides a refreshing escape from the everyday.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 5.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_drink"
],
"tax_rate": 0.08875
},
{
"partner_id": "Western_Omelette",
"name": "Western Omelette",
"description": "The Western Omelette is made with three eggs and filled with diced ham, peppers, onions, and served with home fries and a buttered bagel; it is a classic breakfast dish with a satisfying blend of flavors and textures.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 2,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Linzer_Tart",
"name": "Linzer Tart",
"description": "The Linzer Tart is a traditional Austrian pastry made with a nutty shortbread crust and filled with raspberry preserves, making for a sweet and delicious treat. It's perfect for anyone with a sweet tooth looking for a classic dessert option.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Muffin",
"name": "Muffin",
"description": "A freshly baked and moist muffin, bursting with flavor and perfect for a quick breakfast or snack.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Berry_Blast",
"name": "Berry Blast",
"description": "Berry Blast is a delicious and refreshing smoothie made with a blend of mixed berries, providing a burst of fruity flavor and healthy nutrients in every sip.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "The_Stuy_Towner",
"name": "The Stuy Towner",
"description": "The Stuy Towner smoothie is a delicious blend of creamy peanut butter, ripe banana, and decadent chocolate syrup, making for a satisfying and indulgent treat. Perfect for a quick and tasty breakfast on the go or a midday pick-me-up.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Summer_Love",
"name": "Summer Love",
"description": " is a refreshing blend of sweet strawberries, tropical pineapple, and juicy mango, perfect for a summer day. This drink is sure to transport your taste buds to a tropical paradise with every sip.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Tropicana_Juice",
"name": "Tropicana Juice",
"description": "Tropicana Juice is a refreshing and flavorful beverage made from high-quality fruits that provides essential vitamins and nutrients.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 5.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Banana_split",
"name": "Banana split",
"description": "The Banana Split smoothie is a delightful blend of sweet strawberries, ripe bananas, and rich chocolate syrup, creating a perfectly balanced and indulgent treat.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 3,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "Leo_Omelette",
"name": "Leo Omelette ",
"description": "The Leo Omelette is a savory breakfast dish made with lox, eggs, and onions, served with home fries and a bagel or bialy. It's a classic combination that is both satisfying and delicious.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Create_Your_Own",
"name": "Create Your Own",
"description": "The Create Your Own smoothie allows you to select three toppings of your choice to create a personalized and delicious blend of flavors.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.59,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Turnover",
"name": "Turnover",
"description": "This delectable pastry is a sweet treat that combines soft and sweet apples with a flaky pastry, baked until golden and finished off with a delicious glaze.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Greek_Omelette",
"name": "Greek Omelette",
"description": "The Greek Omelette is a breakfast dish made with 3 eggs, feta cheese, and caramelized onions, served with home fries and a buttered bagel or bialy, and may be a savory option for those looking to start their day with a hearty meal.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 18.6,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Dozen_Fagels",
"name": "Dozen Flat Bagels",
"description": "A dozen of our deliciously chewy flat bagels, perfect for sharing with friends and family for breakfast or brunch. Choose from a variety of flavors including plain, sesame, poppy seed, and everything.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 48.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Eggs_and_Vegetable_O",
"name": "Vegetable Omelette ",
"description": "This dish features a fluffy three-egg omelette filled with nutritious vegetables, accompanied by crispy home fries and a choice of bagel or bialy.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 17.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Croissant",
"name": "Croissant",
"description": "A classic pastry that is perfect for breakfast or a snack, the buttery and flaky croissant can be enjoyed on its own or paired with your choice of butter or jam for added flavor.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Quart_of_Tropicana_J",
"name": "Quart of Tropicana Juice",
"description": "A refreshing and deliciously healthy way to quench your thirst, a quart of Tropicana juice is perfect for sharing with family and friends. Made from 100% pure and natural fruits, it's a great source of vitamins and minerals to help you stay energized throughout the day.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 8.65,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Flagels_49908",
"name": "Flat Bagels",
"description": "Flat bagels are an alternative to the traditional bagel that is thinner and wider with a smaller hole, making it ideal for toasting and sandwich making.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 4.0,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_Danny_Boy_Panini",
"name": "The Danny Boy Panini",
"description": "A culinary masterpiece crafted with care. This delectable creation features a symphony of premium ingredients, perfectly grilled bread, and a harmonious blend of savory and vibrant flavors, delivering a memorable and satisfying experience that will leave you longing for another delightful bite.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "Can_of_Soda",
"name": "Can of Soda",
"description": "\\nQuench your thirst and savor the simple pleasure of an ice-cold can of your favorite soda, offering a crisp and satisfying sip that provides a delightful burst of flavor and a moment of pure refreshment.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 3.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_drink"
],
"tax_rate": 0.08875
},
{
"partner_id": "Cinnamon_Bun_Danish",
"name": "Cinnamon Bun Danish",
"description": "The Cinnamon Bun Danish is a delectable pastry featuring a flaky, buttery dough swirled with cinnamon sugar and drizzled with a sweet glaze, perfect for a breakfast treat or midday snack.",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 6.35,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
},
{
"partner_id": "The_East_Villager",
"name": "The East Villager",
"description": "The East Villager smoothie is a delightful blend of sweet blueberries, tropical pineapple, and creamy banana, creating a perfectly balanced and refreshing drink. It's a great way to start your day or give you a midday energy boost!",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 13.3,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [
"Choose_your_quantity"
],
"tax_rate": 0.08875
},
{
"partner_id": "The_Dylan_Panni",
"name": "The Dylan Panni",
"description": "\\nExperience culinary perfection with The Dylan Panni, a mouthwatering creation that combines layers of succulent meats, cheeses, and fresh ingredients nestled between perfectly grilled bread, offering a harmonious symphony of flavors and textures that will leave you enchanted with every delightful bite.\\n\\n\\n\\n\\n",
"internal_notes": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null,
"media_url": null,
"price": 19.95,
"original_price": 0.0,
"min_amount": 1,
"max_amount": 1,
"partner_availability": null,
"partner_modifiers": [],
"tax_rate": 0.08875
}
],
"modifiers": [
{
"partner_id": "Choose_your_drink",
"name": "Choose your drink",
"is_required": false,
"min_options": 1,
"max_options": 1,
"default_options": [],
"partner_options": [
"Cola",
"Diet-Cola"
],
"type": "variation"
},
{
"partner_id": "Choose_your_quantity",
"name": "Choose your quantity",
"is_required": false,
"min_options": 1,
"max_options": 1,
"default_options": [],
"partner_options": [
"16_oz",
"24_oz"
],
"type": "variation"
}
],
"options": [
{
"partner_id": "24_oz",
"name": "24_oz",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null
},
{
"partner_id": "16_oz",
"name": "16_oz",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null
},
{
"partner_id": "Cola",
"name": "Cola",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null
},
{
"partner_id": "Diet-Cola",
"name": "Diet-Cola",
"price": 0.0,
"original_price": null,
"tax_rate": null,
"description": null,
"is_deactivated": false,
"deactivation_start_date": null,
"deactivation_end_date": null
}
],
"availabilities": []
}